A Complete Guide to PropTypes in React
By John Smith
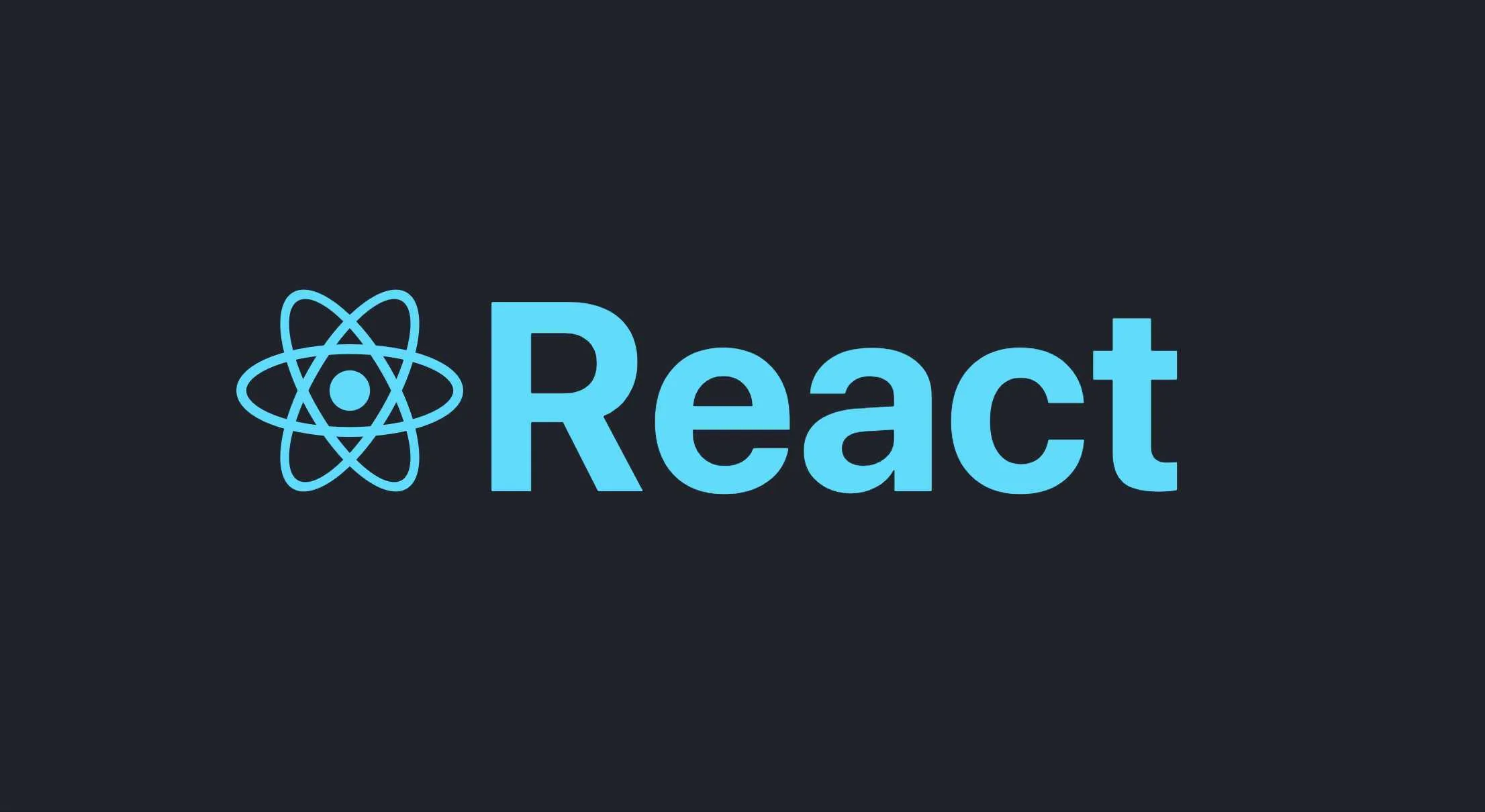
PropTypes is a popular library for type-checking in React that enables developers to validate the types of props passed to a component. In this guide, we will explore how to use PropTypes to validate React component props and provide examples of how to implement it in your React development process.
Table of Contents:
- What are PropTypes in React?
- Why Use PropTypes in React?
- How to Install PropTypes in React
- Validating Props with PropTypes in React
- PropTypes API
- PropTypes Examples
- Summary
- References
- Tips
- FAQs
React is a popular JavaScript library used for building user interfaces. It provides a powerful and flexible framework for creating reusable components that can be combined to build complex applications. One of the key benefits of React is its ability to enforce strict component-based architecture, which improves code organization and makes it easier to maintain and scale applications.
One important aspect of React component development is ensuring that the props passed to a component are of the correct type. This is where PropTypes comes in, a type-checking library that helps you catch errors early in development.
In this guide, we will explore how to use PropTypes to validate React component props and provide examples of how to implement it in your React development process.
What are PropTypes in React?
PropTypes is a type-checking library that is built into React. It provides a way to validate the types of props passed to a component at runtime, which helps catch errors early in the development process. PropTypes can be used to specify the types of props that a component expects, and it will generate warnings in the console if the expected types are not provided.
PropTypes is a simple yet powerful way to validate component props in React. It enables developers to ensure that the props passed to a component are of the correct type and shape, which can help prevent bugs and improve code quality.
Why Use PropTypes in React?
PropTypes provides several benefits for React component development, including:
Catching errors early in development: PropTypes can help catch errors related to incorrect prop types before they cause issues in production.
Improving code quality: By enforcing prop type validation, PropTypes can help ensure that components are used correctly and consistently across an application.
Enhancing code documentation: PropTypes can help document component APIs by clearly specifying the expected types of props that a component accepts.
How to Install PropTypes in React
PropTypes is a built-in library in React, which means that it does not need to be installed separately. However, you will need to import PropTypes from the react package in order to use it.
To import PropTypes, simply add the following line of code at the top of your component file:
import PropTypes from 'prop-types';
This will import the PropTypes library from the react package, making it available for use in your component.
Validating Props with PropTypes in React
PropTypes can be used to validate the types of props that a component expects. To do this, you will need to define the prop types using the PropTypes API.
The PropTypes API provides a variety of validators for different types of data, including strings, numbers, objects, arrays, and more. To use a validator, simply specify the expected type using the corresponding validator method.
For example, to specify that a prop should be a string, you would use the string validator:
PropTypes.string
import React from 'react';
import PropTypes from 'prop-types';
function MyComponent(props) {
return (
<div>
<h1>{props.title}</h1>
<p>{props.text}</p>
</div>
);
}
MyComponent.propTypes = {
title: PropTypes.string.isRequired,
text: PropTypes.string.isRequired,
};
export default MyComponent;
In this example, we have defined a simple component that accepts two props: title and text. We have used the PropTypes.string validator to specify that both props should be strings. We have also added the .isRequired modifier to indicate that the props are required.
If a consumer of this component were to pass in props that do not meet these requirements, PropTypes would generate a warning in the console. For example, if the title prop were passed in as a number instead of a string, PropTypes would generate the following warning:
Warning: Failed prop type: Invalid prop `title` of type `number` supplied to `MyComponent`, expected `string`.
By using PropTypes, we can catch errors like this early in the development process, before they cause issues in production.
PropTypes API
The PropTypes API provides a variety of validators for different types of data. Here are some of the most commonly used validators:
- PropTypes.array: Validates that a prop is an array.
- PropTypes.bool: Validates that a prop is a boolean.
- PropTypes.func: Validates that a prop is a function.
- PropTypes.number: Validates that a prop is a number.
- PropTypes.object: Validates that a prop is an object.
- PropTypes.string: Validates that a prop is a string.
- PropTypes.node: Validates that a prop is a React node.
- PropTypes.element: Validates that a prop is a React element.
- PropTypes.instanceOf: Validates that a prop is an instance of a particular class.
- PropTypes.oneOf: Validates that a prop is one of a specific set of values.
- PropTypes.oneOfType: Validates that a prop is one of a specific set of types.
- PropTypes.arrayOf: Validates that a prop is an array of a specific type.
- PropTypes.objectOf: Validates that a prop is an object with a specific value type.
- PropTypes.shape: Validates that a prop is an object with specific shape.
For a complete list of validators and their usage, see the PropTypes documentation on the React website.
PropTypes Examples
Here are some examples of how to use PropTypes to validate component props in React:import React from 'react';
import PropTypes from 'prop-types';
function MyComponent(props) {
return (
<div>
<h1>{props.title}</h1>
<p>{props.text}</p>
{props.children}
</div>
);
}
MyComponent.propTypes = {
title: PropTypes.string.isRequired,
text: PropTypes.string,
children: PropTypes.element.isRequired,
};
export default MyComponent;
In this example, we have defined a component that accepts three props: title, text, and children. We have used PropTypes.string to specify that title should be a string and PropTypes.element to specify that children should be a React element. We have also made title a required prop by adding the .isRequired modifier.
Note that we have not made text a required prop. This means that if text is not passed in as a prop, PropTypes will not generate a warning.
Here's another example that uses the PropTypes.oneOfType
import React from 'react';
import PropTypes from 'prop-types';
function MyComponent(props) {
return (
<div>
<h1>{props.title}</h1>
{props.subtitle && <h2>{props.subtitle}</h2>}
<p>{props.text}</p>
</div>
);
}
MyComponent.propTypes = {
title: PropTypes.string.isRequired,
subtitle: PropTypes.oneOfType([PropTypes.string, PropTypes.number]),
text: PropTypes.string.isRequired,
};
export default MyComponent;
In this example, we have defined a component that accepts three props: title, subtitle, and text. We have used PropTypes.string to specify that title and text should be strings. We have also used PropTypes.oneOfType to specify that subtitle can be either a string or a number.
Note that we have used the && operator to conditionally render the subtitle prop. This is a common pattern for rendering optional props in React.
Summary
PropTypes is a powerful library for type-checking in React that enables developers to validate the types of props passed to a component. By using PropTypes, you can catch errors early in development, improve code quality, and enhance code documentation.
To use PropTypes in your React component development process, simply import PropTypes from the react package and define the prop types using the PropTypes API. You can use a variety of validators to specify the expected types of props, including PropTypes.string, PropTypes.number, PropTypes.bool, and more.
By using PropTypes, you can ensure that your React components are used correctly and consistently across your application.
References
- PropTypes documentation: https://reactjs.org/docs/typechecking-with-proptypes.html
Tips
- Always validate your component props using PropTypes to catch errors early in development.
- Use the .isRequired modifier to indicate that a prop is required.
- Use conditional rendering to handle optional props in your component.
- Document your component APIs using PropTypes to improve code documentation.
FAQs
Q: Can PropTypes be used with functional components in React?
A: Yes, PropTypes can be used with both class and functional components in React.
Q: Can PropTypes validate the shape of an object prop?
A: Yes, you can use the PropTypes.shape validator to validate the shape of an object prop. For example:
MyComponent.propTypes = {
person: PropTypes.shape({
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
}).isRequired,
};
This will ensure that the person prop is an object with a name property that is a string and an age property that is a number.
Q: Can PropTypes be used for validating props passed to child components?
A: Yes, PropTypes can be used to validate props passed to child components. You can define PropTypes for the props passed to a child component just as you would for props passed to a parent component.
For example, consider the following parent component:
import React from 'react';
import PropTypes from 'prop-types';
import ChildComponent from './ChildComponent';
function ParentComponent(props) {
return (
<div>
<h1>{props.title}</h1>
<ChildComponent name={props.name} age={props.age} />
</div>
);
}
ParentComponent.propTypes = {
title: PropTypes.string.isRequired,
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
export default ParentComponent;
In this example, we have defined a parent component that passes name and age props to a child component. We have used PropTypes to validate the types of these props, just as we would for props passed to the parent component.
Now consider the following child component:
import React from 'react';
import PropTypes from 'prop-types';
function ChildComponent(props) {
return (
<div>
<h2>{props.name}</h2>
<p>{props.age}</p>
</div>
);
}
ChildComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
export default ChildComponent;
In this example, we have defined a child component that accepts name and age props. We have used PropTypes to validate the types of these props, just as we did for the parent component.
By using PropTypes to validate props passed to child components, you can catch errors early in development and ensure that your components are used correctly and consistently across your application.
Q: Can PropTypes be used for validating props passed through Redux or other state management libraries?
A: Yes, PropTypes can be used to validate props passed through Redux or other state management libraries. In fact, it is a good practice to validate the types of props passed through these libraries to ensure that they are used correctly and consistently throughout your application.
To use PropTypes with Redux or other state management libraries, simply define PropTypes for the props passed to your components, just as you would for props passed directly to your components. You can use the PropTypes library to validate the types of props passed through your state management library, just as you would for any other props.
For example, consider the following Redux-connected component:
import React from 'react';
import { connect } from 'react-redux';
import PropTypes from 'prop-types';
function MyComponent(props) {
return (
<div>
<h1>{props.title}</h1>
<p>{props.text}</p>
</div>
);
}
MyComponent.propTypes = {
title: PropTypes.string.isRequired,
text: PropTypes.string.isRequired,
};
function mapStateToProps(state) {
return {
title: state.title,
text: state.text,
};
}
export default connect(mapStateToProps)(MyComponent);
In this example, we have defined a Redux-connected component that uses mapStateToProps to map state to props. We have used PropTypes to validate the types of the props passed to the component, just as we would for any other component.
By using PropTypes with Redux or other state management libraries, you can ensure that your components are used correctly and consistently throughout your application, improving code quality and preventing errors.